创建基本的web应用
SpringBoot可以快速的创建一个restful的web应用。下面开始从零开始创建一套基本的web应用。
使用idea创建Spring initializr
我这里直接使用idea中的Spring initializr
来创建SpringBoot应用。同理可以使用start.spring.io中。创建完成后直接在IDE中导入即可。
- 需要在idea中新建项目
- 填写项目的基本信息
- 勾选需要的依赖
- 最后点击finish完成创建。
文档结构说明
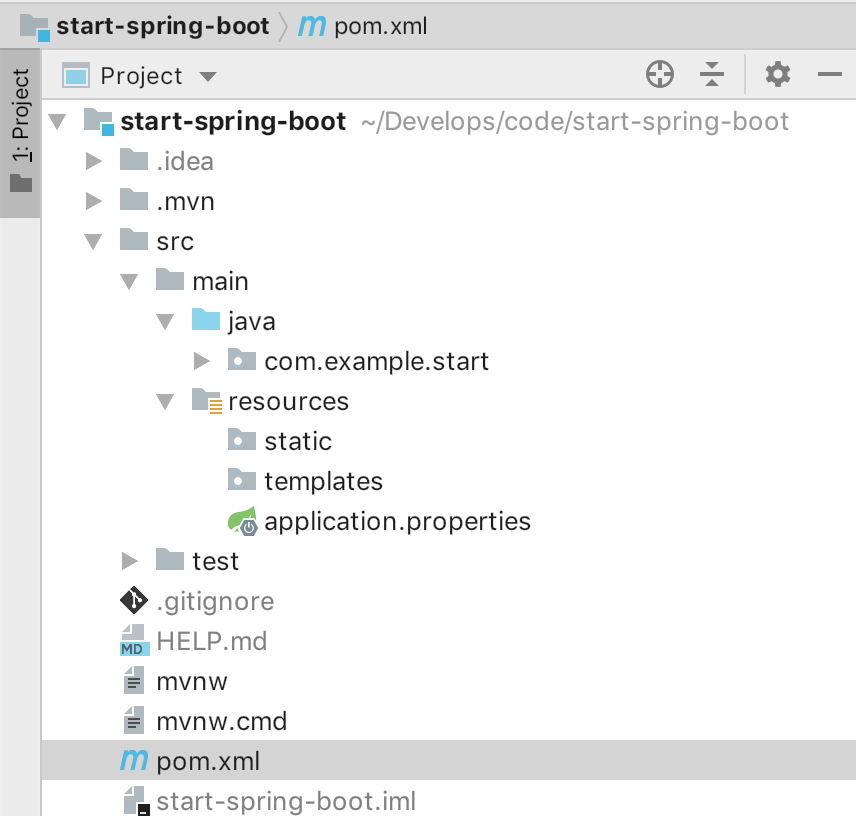
新建SpringBoot应用后自动创建的目录结构如如上图,其中SpringBoot启动类StartSpringBootApplication
放在java/com/example/start
文件夹中,用于启动整个项目。
对于资源文件,SpringBoot给出来一套目录标准。
resource文件夹中的application.properteis为整体的SpringBoot配置文件。其中有static和templates目录。
- static 文件夹用于存放静态资源,如js,css
- templates 文件夹用于存放模版文件,如jsp,html
那么SpringBoot是如何建立这些目录文件标准的呢?
web目录相关的自动配置
web目录相关的自动配置是在WebMvcAutoConfiguration
自动配置类中进行配置。而对资源的加载使用的是addResourceHandler
方法
1 |
|
webjars
WebJars是打包到JAR(Java Archive)文件中的客户端Web库(例如jQuery和Bootstrap)。
from webjars官网
根据以上内容举个例子来讲解如何使用webjars进行静态资源的加载:
- 去官网找相关资源的依赖
- 添加到pom.xml文件中
以粉色的竖线为分割线,左侧为下载完的webjars的jar包,右侧为在pom.xml文件中的依赖信息。
加载webjars
如果SpringBoot发现有webjar的应用jar包,则加载路径classpath:/META-INF/resources/webjars/
下的文件。在图中例子中就是jquery
文件夹下的所有内容。这样SpringBoot就能够识别jquery包下的所有静态文件了。
映射webjars
SpringBoot会将所有已将加载jars映射到 /webjars/**
这个路径上。
比如上面我们添加了jquery.min.js。我们启动程序,可以在http://localhost:8080/webjars/jquery/3.4.1/jquery.min.js
这个地址上访问,发现系统已经通过webjars将资源加载到了。在实际的使用中,使用script标签引用webjars也是引用这个地址的静态文件。
项目内静态资源
使用webjars用来加载静态资源非常方便,但是如果自己写的js和css等静态资源也需要加载时,就需要放到SpringBoot默认加载的目录下。
1 | "spring.resources", ignoreUnknownFields = false) (prefix = |
通过getStaticLocations获取静态资源的目录,默认的SpringBoot定义了classpath下的以下的文件,都可以放置静态文件,都可以通过url的方式访问到这个静态文件。
classpath:/META-INF/resources
classpath:/resources/
classpath:/static/
classpath:/public/
需要注意的是这个classpath就是一开始创建SpringBoot应用帮我们创建好的resource路径
这个类也是一个xxxxProperties
类,所以可以通过改变相应的配置文件中的属性来改变这个路径。这里的路径使用的变量是staticLocations
,所以相应的在application.properties文件中可以使用spring.resources.staticLocations=路径名称
就行了。
主页的设置
SpringBoot默认的使用静态资源文件夹下的index.html
作为首页。
1 | private Optional<Resource> getWelcomePage() { |
如果找到主页的index.html后,就开始做主页的映射操作
1 |
|
上面是依据SpringBoot加载静态资源的方式做的简单总结。
添加controller
在上一步创建完项目后,可以紧跟着写一个SpringMVC测试的controller,看看能否启动项目。
1 | // 起到了@Controller和@Responsebody两种作用,实际上就是 |
如果你也像我勾选来数据库相关的starters,在启动时,控制台会提示需要配置数据库的基本配置。
那么怎么找这些配置呢?
- 去官网找配置
- 直接看代码
如果你没有碰到上面这个问题,那么请忽略以下部分。
因为前几篇文章中已经分析来SpringBoot是如何完成自动配置的,所以这里我想通过找代码的方式直接看它能够配置哪些属性。
- 首先在
autoconfiguration
包下面的spring.factory中搜索jdbc
- 点击关于DataSource的自动配置类
1 | (DataSourceProperties.class) |
根据DataSourceProperties类,可以知道如果我们像配置DataSource,那么有这几个属性可以进行配置。
1 | # spring.datasource 为前缀,后面为属性名,和Properties类中的属性相对应。 |